开发过程中经常会遇到参数配置化的情景,参数的类型也可以是多种多样的,记录一下各类型从配置文件中的加载方式,给当前创建一个savepoint。
配置一个properties文件
1 2 3 4
| > cc.common=abcdef > cc.list=a,b,c,d,e,f > cc.map={key1: '1', key2: '2', key3: '3'} >
|
基础案例
1. 字符串
1 2 3 4 5 6 7
| @Value("${cc.common}") private String common;
@Value("${cc.common1:aaa}") private String commonDefault;
|
2. 数组
1 2
| @Value("${cc.list}") private String[] array;
|
3. List
1 2
| @Value("#{'${cc.list}'.split(',')}") private List<String> list;
|
3. Map
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| @Value("#{${cc.map}}") private Map<String, Integer> map;
@Value("#{${cc.map}.key1}") private Integer value;
@Value("#{${cc.map}.?[value>'1']}") private Map<String, Integer> mapFilter;
@Value("#{${cc.map:{key1: '11', key2: '12'}}}") private Map<String, Integer> map;
|
4. 自定义对象
1 2 3 4 5 6
| @ConfigurationProperties(prefix = "cc") @Data public class PropertiesInject { private String common; private String[] list; }
|
5. 项目变量
1 2 3 4 5 6 7 8 9 10 11
| @Value("#{@systemProperties}") private String systemProperty;
@Value("#{@systemProperties['user.dir']}") private String systemPropertyList;
@Value("#{@systemProperties}") private Map<String, String> systemPropertyMap;
|
6. 系统环境变量
1 2 3 4 5 6 7 8 9 10 11
| @Value("#{@systemEnvironment}") private Map<String, String> systemEnvironment;
@Value("#{@systemEnvironment['PATH']}") private String systemEnvironmentPath;
@Value("#{@systemEnvironment['abc']?:'snowman'}") private String systemEnvironmentDefault;
|
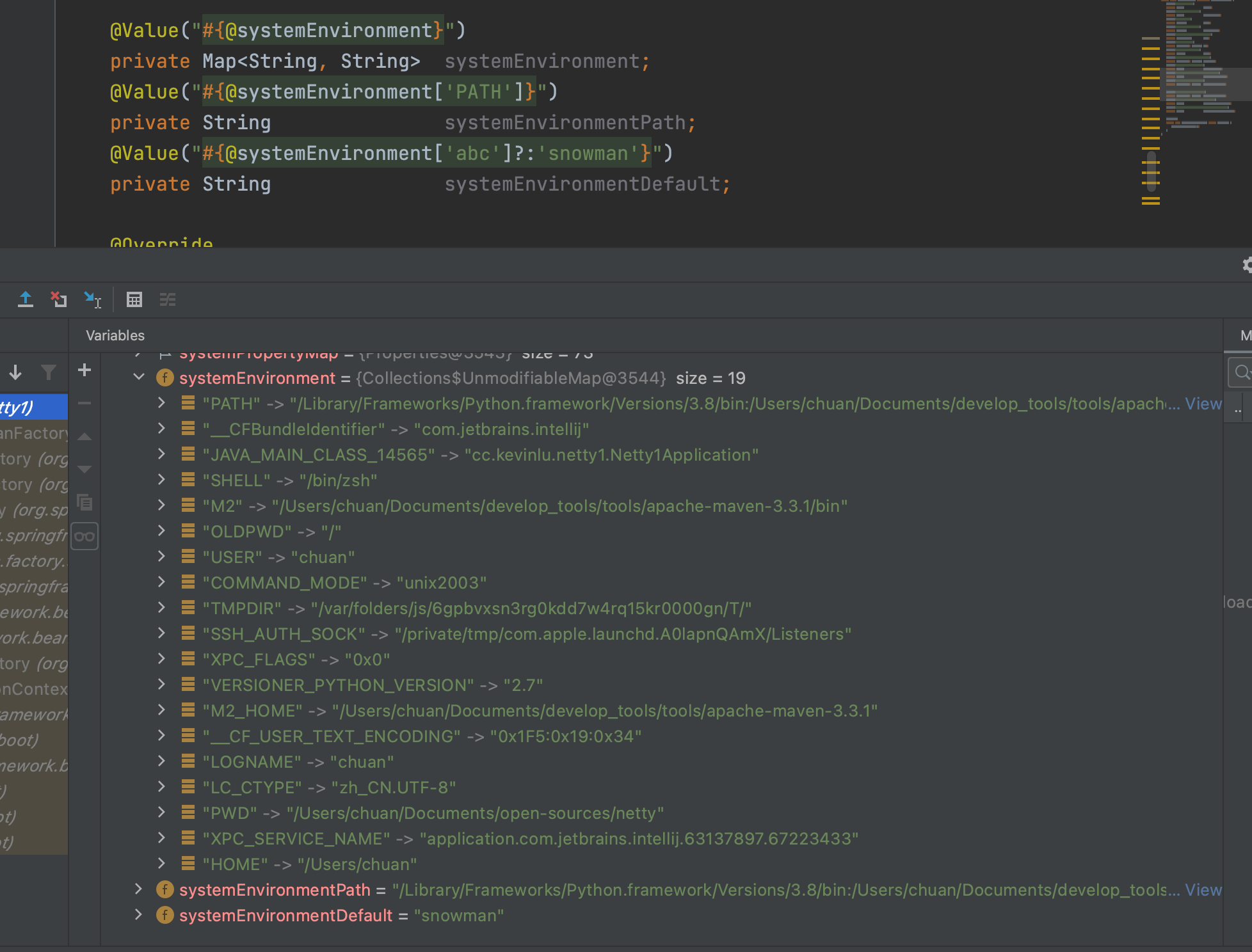
注入方式
1. 属性注入
就是上方案例中的注入方式
2. 构造器注入
1 2 3 4 5 6 7 8 9 10 11 12
| @Component @PropertySource("classpath:values.properties") public class PropertiesInject {
private List<String> values = new ArrayList<>();
@Autowired public void setValues(@Value("#{'${cc.list}'.split(',')}") List<String> values) { this.values.addAll(values); }
}
|
3. Setter注入
1 2 3 4 5 6 7 8 9 10 11 12
| @Component @PropertySource("classpath:values.properties") public class PropertiesInject {
private List<String> values = new ArrayList<>();
@Autowired public void setValues(@Value("#{'${cc.list}'.split(',')}") List<String> values) { this.values.addAll(values); }
}
|
4. 类统一注入
1 2 3 4 5 6
| @ConfigurationProperties(prefix = "cc") @Data public class PropertiesInject { private String common; private String[] list; }
|